Eloquent is Laravel’s built-in Object-Relational Mapper (ORM) that provides a simple and efficient way to interact with your database. How to use Eloquent, It allows you to work with database tables as if they were PHP objects and is known for its clean and expressive syntax. Here’s a guide to help you get started with Eloquent.
How to use Eloquent Example
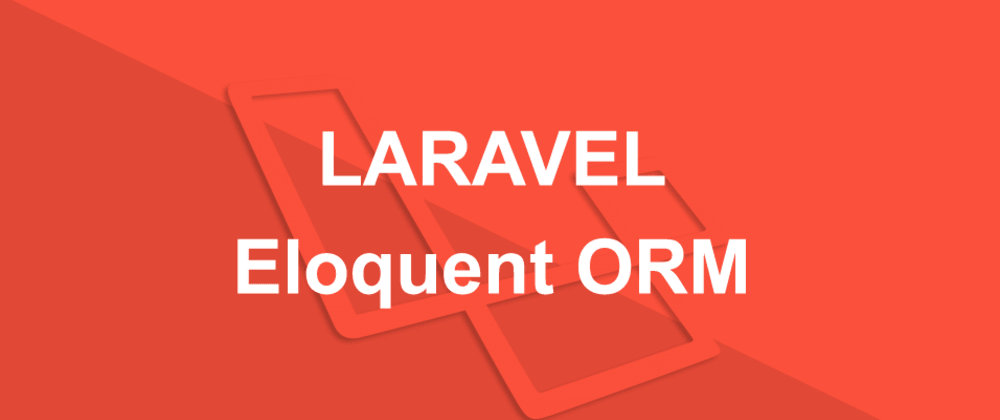
Steps for How to use Eloquent?
1. Setting Up Models
Each Eloquent model corresponds to a database table, and each instance of the model represents a row in that table.
a. Creating a Model
You can create a model using the artisan command:
php artisan make:model Post
This will create a Post
model in the app/Models
directory (or app/
for older Laravel versions).
b. Model-Table Conventions
Eloquent assumes some conventions:
- Table names are plural (e.g.,
posts
for thePost
model). - Primary keys are named
id
by default.
You can override these conventions if necessary.
2. Defining a Model
Here’s an example of a simple Post
model that interacts with the posts
table:
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Post extends Model
{
// If your table name differs from the convention
protected $table = 'posts';
// Define which fields can be mass-assigned
protected $fillable = ['title', 'body'];
}
$table
: Specifies the table name.$fillable
: Defines the attributes that can be mass-assigned.
3. Basic CRUD Operations
a. Create Records
To insert a new record into the database, you can create a new instance of the model, set its properties, and save it. You Can Learn How to deploying Laravel projects on a live server – Complete Step-by-Step Guide
$post = new Post();
$post->title = "My First Post";
$post->body = "This is the content of the post";
$post->save();
Alternatively, you can use the create
method if you have defined the fillable
array:
Post::create([
'title' => 'My Second Post',
'body' => 'This is another post content',
]);
b. Read Records
You can fetch records from the database using Eloquent’s query methods.
- Retrieve all records:
$posts = Post::all();
Find a record by ID:
$post = Post::find(1);
Query with conditions:
$posts = Post::where('title', 'like', '%First%')->get();
Retrieve the first match:
$post = Post::where('title', 'My First Post')->first();
Update Records
To update a record, first retrieve it, modify its attributes, and call save()
:
$post = Post::find(1);
$post->title = "Updated Title";
$post->save();
Alternatively, use the update
method:
Post::where('id', 1)->update(['title' => 'Updated Title']);
Delete Records
To delete a record:
$post = Post::find(1);
$post->delete();
Or use the destroy
method for bulk deletion:
Post::destroy([1, 2, 3]); // Deletes records with IDs 1, 2, and 3
4. Eloquent Relationships
Eloquent makes it easy to define relationships between models. Here are some of the common types:
a. One-to-One
Example: A user has one profile.
class User extends Model
{
public function profile()
{
return $this->hasOne(Profile::class);
}
}
b. One-to-Many
Example: A post has many comments.
class Post extends Model
{
public function comments()
{
return $this->hasMany(Comment::class);
}
}
c. Many-to-Many
Example: A user belongs to many roles, and a role belongs to many users.
class User extends Model
{
public function roles()
{
return $this->belongsToMany(Role::class);
}
}
d. Inverse Relationships
Eloquent also supports inverse relationships. For instance, if a comment belongs to a post:
class Comment extends Model
{
public function post()
{
return $this->belongsTo(Post::class);
}
}
5. Query Scopes
You can define reusable query logic by using scopes. Scopes allow you to encapsulate commonly used query constraints.
a. Local Scopes
Define a local scope in the model:
class Post extends Model
{
public function scopePublished($query)
{
return $query->where('published', true);
}
}
Use it in a query like this:
$publishedPosts = Post::published()->get();
b. Global Scopes
Global scopes apply a query constraint to all queries for a model. For example, if you want to always return only active posts:
class ActiveScope implements \Illuminate\Database\Eloquent\Scope
{
public function apply(\Illuminate\Database\Eloquent\Builder $builder, Model $model)
{
$builder->where('status', 'active');
}
}
// In the model
protected static function booted()
{
static::addGlobalScope(new ActiveScope);
}
6. Eager Loading (Avoiding N+1 Query Problem)
Eloquent supports eager loading to load related models efficiently:
$posts = Post::with('comments')->get(); // Loads posts with their comments
7. Soft Deleting
Soft deleting allows you to “delete” a record without actually removing it from the database. Laravel provides this feature out of the box.
a. Enable Soft Deleting
Add the SoftDeletes
trait to your model:
use Illuminate\Database\Eloquent\SoftDeletes;
class Post extends Model
{
use SoftDeletes;
protected $dates = ['deleted_at'];
}
Now when you call delete()
, the record is not removed from the database but instead marked as deleted by setting the deleted_at
column.
b. Restoring Soft Deleted Records
You can restore soft deleted records:
$post = Post::withTrashed()->find(1);
$post->restore();
8. Accessors and Mutators
Eloquent allows you to modify how attributes are accessed or mutated (stored) in the database. You Can Learn How to integrate google Spreadsheet in laravel 11
a. Accessors
For example, if you want to ensure that a post’s title is always capitalized when accessed:
class Post extends Model
{
public function getTitleAttribute($value)
{
return ucfirst($value);
}
}
b. Mutators
To modify how data is saved to the database, you can use mutators:
class Post extends Model
{
public function setTitleAttribute($value)
{
$this->attributes['title'] = strtolower($value);
}
}
Conclusion
Eloquent simplifies interacting with databases by turning your data into clean, readable models. Mastering CRUD operations, relationships, query scopes, and other advanced features like eager loading, soft deletes, and accessors/mutators will give you a lot of power over your application’s data layer. You Can See Laravel Eloquent Docs